Maximizing Python Time Effeciency
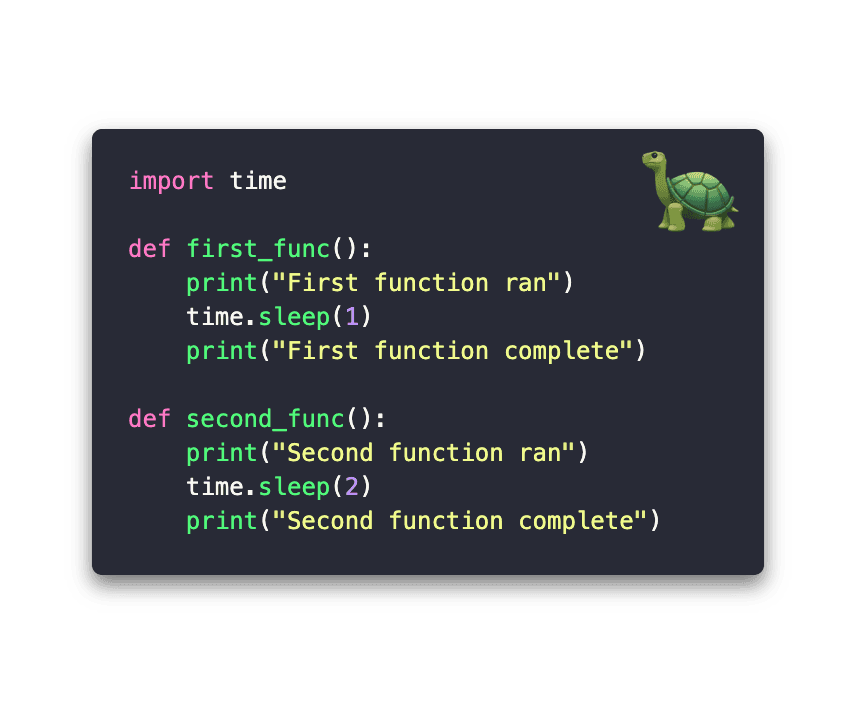
Simple Example
One of the ways to maximize efficiency in Python is by employing multi-threading to optimize tasks that can be performed in parallel. This can significantly reduce the time taken to complete certain operations.
Let's take a look at a simple example:
import time
def first_func():
print("First function ran")
time.sleep(1)
print("First function complete")
def second_func():
print("Second function ran")
time.sleep(2)
print("Second function complete")
first_func()
second_func()
When run sequentially, this script prints a message, waits for a set amount of time (simulating a task), and then prints a completion message. Running this code will take approximately 3 seconds - the sum of the sleep times.
This is because the second function only starts after the first function has completed.
Optimizing for Time Effeciency
But what if we could execute both functions simultaneously? This is where threading comes into play. Let's take a look at how we can optimize this process:
from threading import Thread
## previous code
thread1 = Thread(target=first_func)
thread2 = Thread(target=second_func)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
By using the Thread module from Python's threading library, we can run first_func() and second_func() in separate threads. The start() method initiates each thread, and the join() method ensures that the main program waits for all threads to complete before continuing.
Optimized Result
import time
from threading import Thread
def first_func():
print("First function ran")
time.sleep(1)
print("First function complete")
def second_func():
print("Second function ran")
time.sleep(2)
print("Second function complete")
thread1 = Thread(target=first_func)
thread2 = Thread(target=second_func)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
Running this script will start both first_func() and second_func() almost simultaneously in separate threads, thereby reducing the total execution time.